Thermistor Interfacing with Arduino UNO
Introduction
Thermistor is a variable resistance element, whose resistance varies with change in temperature. The change in resistance value is a measure of the temperature.
Thermistors are classified as PTC (Positive Temperature Coefficient) or NTC (Negative Temperature Coefficient).
They can be used as current limiters, temperature sensors, overcurrent protectors etc.
For more information about thermistor and how to use it, refer the topic NTC Thermistor in the sensors and modules section.
Interfacing Diagram
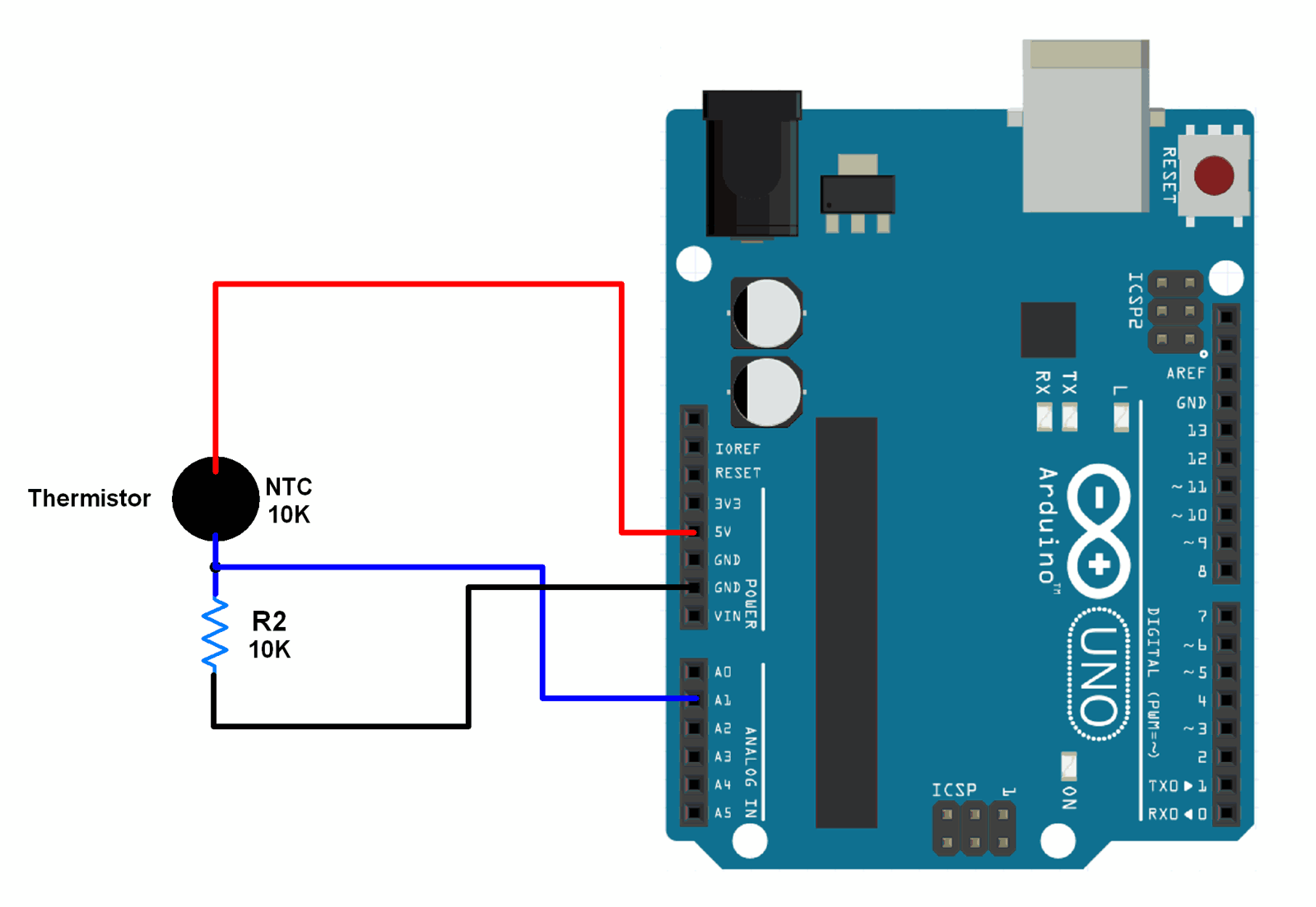
Example
Measuring temperature using thermistor.
Here, an NTC type thermistor of 10kΩ is used. NTC of 10kΩ means that this thermistor has a resistance of 10kΩ at 25°C.
Voltage across the 10kΩ resistor is given to the ADC of UNO board.
The thermistor resistance is found out using simple voltage divider network formula.
Rth is the resistance of thermistor
Vout is the voltage measured by the ADC
The temperature can be found out from thermistor resistance using the Steinhart-Hart equation.
Temperature in Kelvin = 1 / (A + B[ln(R)] + C[ln(R)]^3)
where A = 0.001129148, B = 0.000234125 and C = 8.76741*10^-8
and R is the thermistor resistance.
Sketch For Temperature Measurement Using Thermistor
#include <math.h>
const int thermistor_output = A1;
void setup() {
Serial.begin(9600); /* Define baud rate for serial communication */
}
void loop() {
int thermistor_adc_val;
double output_voltage, thermistor_resistance, therm_res_ln, temperature;
thermistor_adc_val = analogRead(thermistor_output);
output_voltage = ( (thermistor_adc_val * 5.0) / 1023.0 );
thermistor_resistance = ( ( 5 * ( 10.0 / output_voltage ) ) - 10 ); /* Resistance in kilo ohms */
thermistor_resistance = thermistor_resistance * 1000 ; /* Resistance in ohms */
therm_res_ln = log(thermistor_resistance);
/* Steinhart-Hart Thermistor Equation: */
/* Temperature in Kelvin = 1 / (A + B[ln(R)] + C[ln(R)]^3) */
/* where A = 0.001129148, B = 0.000234125 and C = 8.76741*10^-8 */
temperature = ( 1 / ( 0.001129148 + ( 0.000234125 * therm_res_ln ) + ( 0.0000000876741 * therm_res_ln * therm_res_ln * therm_res_ln ) ) ); /* Temperature in Kelvin */
temperature = temperature - 273.15; /* Temperature in degree Celsius */
Serial.print("Temperature in degree Celsius = ");
Serial.print(temperature);
Serial.print("\t\t");
Serial.print("Resistance in ohms = ");
Serial.print(thermistor_resistance);
Serial.print("\n\n");
delay(1000);
}
No comments:
Post a Comment